本記事は元記事の日本語訳です。
Android TV アプリ開発入門
Nexus Player, SONY BRAVIAと、Android TV(アンドロイドテレビ)が発売されてからすでに時間がたっているがまだまだAndroid TVアプリの開発に関する情報は少ない。そこでここでは、Android TVアプリを実際に自分で実装しながら学べる形式の開発チュートリアルを書いていければと思う。
本稿ではAndroid “TV”プラットフォームに特化した実装を主に紹介していく。特にUIの実装がメイン、なぜならUI はスマホとテレビではその基本思想が大きく異なるからだ。たとえばテレビはリモコンで気軽に操作するようにできているので、 ↑↓→← といった上下左右の方向キーだけで(タッチを使わずに)”気軽に”操作できるようなUIが要求される。
こういったユーザーが”気軽に”操作できるようにするための基礎プラットフォームとしてAndroid open source project(AOSP)からLeanback Support library (android.support.v17.leanback
) が提供されている。ちなみにここでいうLeanback(リーンバック)とは、”後ろにもたれる”というような意味。ソファーにもたれながらでも、TVを簡単に操作できるようなUIが理想ですよーという事だろう。
このLeanback Support libraryを使ってアプリを開発することで、このようなUI思想に基づいたアプリが作れるというわけだ。ということで、本稿では主にこのLeanback libraryの使い方を紹介していく。
想定する読者層
- Androidアプリは開発したことがあるが、Android TVアプリに関しては何も知らない
- 初心者~中級者向け
使用するエディタ
Eclipseは2015年にサポートが終わっているので、 Android studio を使って開発を進めていく。Androidアプリ開発では今後標準となるIDEでとてもつかいやすいので、まだ使ったことのない方は、ここからAndroid Studioをダウンロードして設定しましょう!ちなみに、自分は英語版のAndroid Studioをそのまま使っているので日本語版の方は適宜読み替えて読み進めていただければと思います。
注:なお、本稿で説明するソースはAOSPで公開されている android TV サンプルソースコードが元ネタで、ただこのソースコードをもとに、より細かく説明しているだけです。
新しいAndroid TV アプリプロジェクトの作成
Android studio
起動
New project
Application name: AndroidTVappTutorial
Company Domain: corochann.com ( or you can use your own domain name )
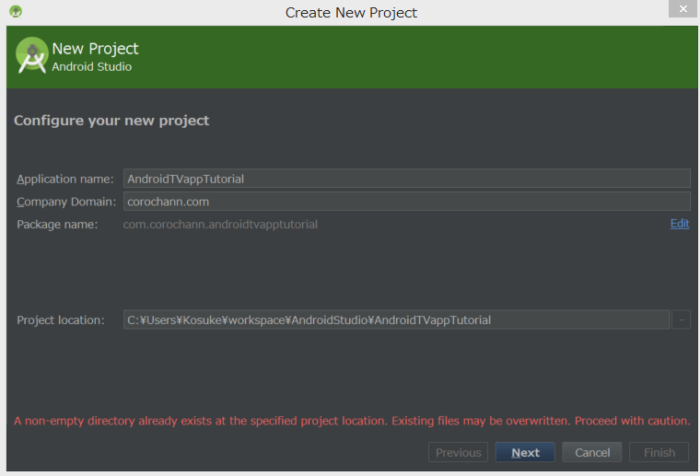
Target Android Devices
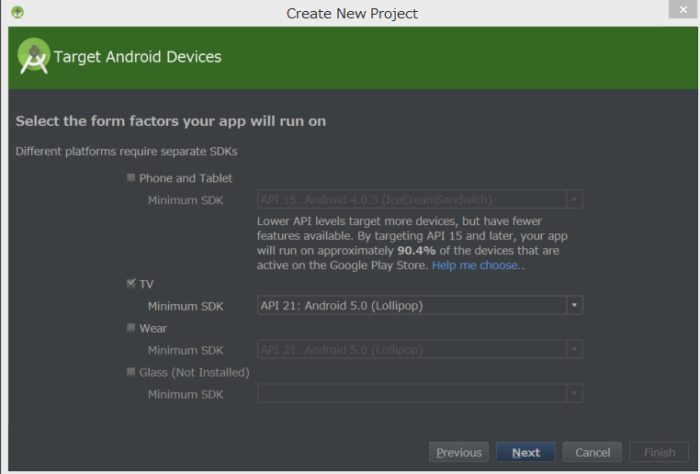
Add an activity to TV
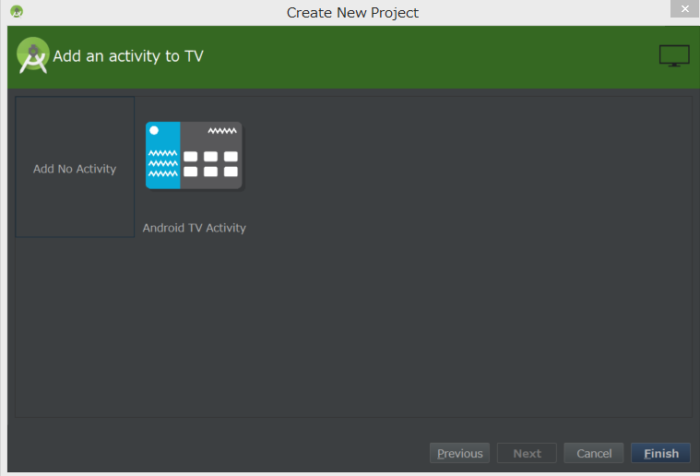
“Add No Activity” 選択後、Finish
するとAndroid studio が自動でAndroidアプリ開発を進めるにあたって必要な最低限のソースコードを自動生成してくれる。
ここまでの手順が終わった状態のソースコードは githubで。
- Add activity
はじめにActivityを作成する。 “com.corochann.androidtvapptutorial”を右クリックして
New -> Activity -> Blank activity
“Launcher Activity”をチェック。
そして”MainActivity
“という名前で、blank activityを作成する。
すると、Android studioはJava class と layout/activity_main.xmlを自動生成してくれる(res/menu/menu_main.xmlも作成されるが、Android TVアプリでは使わないので無視)
* 注: Android Studio で、”Android TV activity” という項目も見つけることができる。これを選択すると一気に大量のファイルが自動生成される。Leanbackライブラリの各種機能がサンプル実装された状態でのファイルが生成されるので、参考ファイルとしては役立つんだけれど、一度に大量のファイルが生成されるので、どのファイルがどの機能とつながっているのかいまいちわかりづらい。
ということで、本稿ではこれらの機能を一つずつ実装していくことによって、理解を進めていきたい。
次に MainActivity の 実際のUI 表示部分を担当する MainFragmentを作る。
- Add fragment
パッケージネームを右クリック (例 com.corochann.androidtvapptutorial)
New -> Java Class -> Name: MainFragment
* 上の手順の代わりに、New -> Fragment -> Blank fragment Uncheck “Create layout XML?” を選択すると、これまた一気に不要なコードまで自動生成されてしまうので、今回は空のJava ClassからFragmentを作成していく。
まず、 activity_main.xml を以下のように変更して、MainActivity
がMainFragment
のみを表示するようにする。
* 以後、ソースのコピペをしたいときは、右上の “<>” アイコンをクリックするとできます。
<?xml version="1.0" encoding="utf-8"?> <fragment xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/main_browse_fragment" android:name="com.corochann.androidtvapptutorial.MainFragment" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity" tools:deviceIds="tv" tools:ignore="MergeRootFrame" />
次に MainFragment
の修正
この MainFragment
を BrowseFragment
のサブクラスとする。BrowseFragment class はAndroid SDK Leanback libraryで提供されているクラスで、Android TV の基本・標準となるUIを構築するために使われる(すぐ後、次の章で実例が表示されます)。
package com.corochann.helloandroidtvfromscrach; import android.os.Bundle; import android.support.v17.leanback.app.BrowseFragment; import android.util.Log; public class MainFragment extends BrowseFragment { private static final String TAG = MainFragment.class.getSimpleName(); @Override public void onActivityCreated(Bundle savedInstanceState) { Log.i(TAG, "onActivityCreated"); super.onActivityCreated(savedInstanceState); } }
(I) ビルド・実行
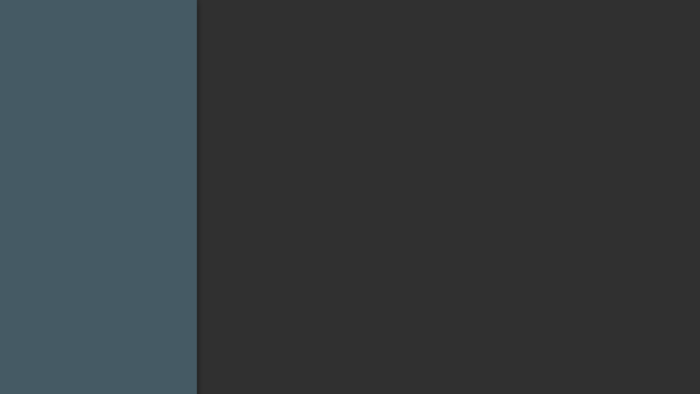
BrowseFragment
の構成要素である HeadersFragment
と RowsFragment
が確認できる。HeaderFragment
(ヘッダー) が右側、 RowsFragment
(コンテンツ部分) が左側。このフラグメントの基本設定を行っていく。
まずは初めにBrowseFragment
のメインカラーとタイトルを設定しよう。
ここまでのソースコード: github
- setupUIElements() をMainFragment.javaに実装
MainFragment.javaにsetupUIElements()
を実装する、このメソッドはアプリの基本UI情報を設定する。
@Override public void onActivityCreated(Bundle savedInstanceState) { Log.i(TAG, "onActivityCreated"); super.onActivityCreated(savedInstanceState); setupUIElements(); } private void setupUIElements() { // setBadgeDrawable(getActivity().getResources().getDrawable(R.drawable.videos_by_google_banner)); setTitle("Hello Android TV!"); // Badge, when set, takes precedent // over title setHeadersState(HEADERS_ENABLED); setHeadersTransitionOnBackEnabled(true); // set fastLane (or headers) background color setBrandColor(getResources().getColor(R.color.fastlane_background)); // set search icon color setSearchAffordanceColor(getResources().getColor(R.color.search_opaque)); }
ここで設定しているのは
- アプリタイトル もしくは アプリアイコン
- ブランドカラー
色情報は colors.xml から参照される。このファイルを作成しよう。res/values を右クリックして、
New -> values Resource file
File name: colors.xml -> “OK”
と選択。できたファイルに以下のように記載する。
<?xml version="1.0" encoding="utf-8"?> <resources> <color name="fastlane_background">#0096e6</color> <color name="search_opaque">#ffaa3f</color> </resources>
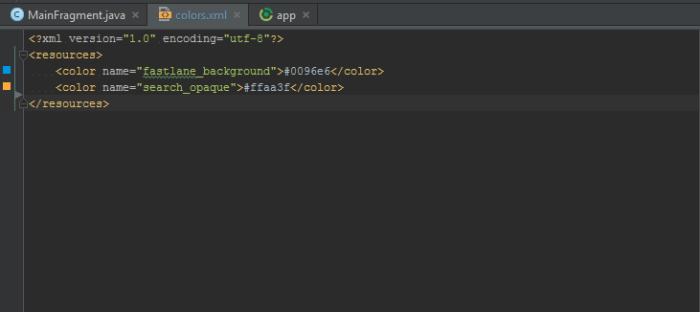
(II) ビルド・実行
右上にタイトルが表示され、ヘッダー部分の色がブランドカラーで指定した色になっていればOK。
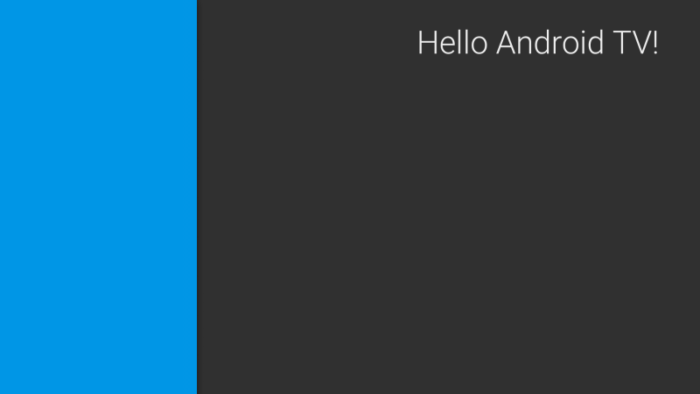
ちなみに、 setTitle()
のかわりに setBadgeDrawable()
を使うこともできる。こちらのメソッドを用いた時にはタイトルテキストの代わりにアイコンが表示される。また、両方のメソッドを読んだ場合にはアイコン表示が優先される。
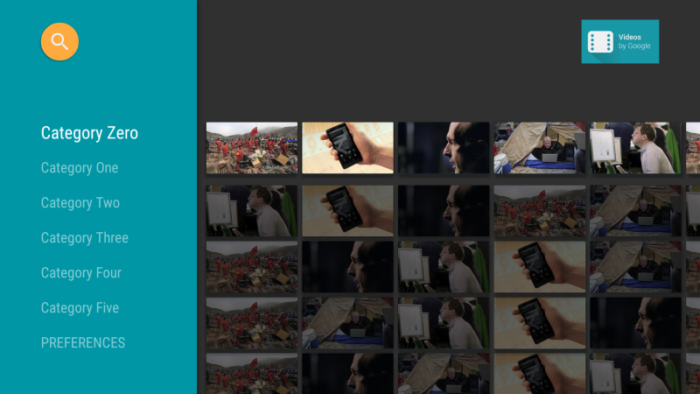
ここまでのソースコード: github.
- Android Manifest の修正
ここまでのソースコードでは、アプリのアイコンが Leanback Launcher (Android TV のホーム画面・ホームランチャーアプリ) に表示されていなかったと思う。
アプリのランチャー(起動用)アイコンをAndroid TVで表示するためには、 AndroidManifest.xml を以下のようにする。
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.corochann.androidtvapptutorial" > <!-- TV app need to declare touchscreen not required --> <uses-feature android:name="android.hardware.touchscreen" android:required="false" /> <!-- true: your app runs on only TV false: your app runs on phone and TV --> <uses-feature android:name="android.software.leanback" android:required="true" /> <application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:theme="@style/Theme.Leanback" > <activity android:name=".MainActivity" android:icon="@drawable/app_icon_your_company" android:label="@string/app_name" android:logo="@drawable/app_icon_your_company" > <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LEANBACK_LAUNCHER" /> </intent-filter> </activity> </application> </manifest>
また、 app_icon_your_compan.png は main/res/drawble/ フォルダの中に入れた。
(III) ビルド・実行
Leanback launcherにてアプリのランチャーアイコンを表示するには以下の宣言を行う必要がある。
<category android:name="android.intent.category.LEANBACK_LAUNCHER" />
またアイコン・ロゴはActivityタグ内で指定されている。
<activity android:name=".MainActivity" android:icon="@drawable/app_icon_your_company" android:label="@string/app_name" android:logo="@drawable/app_icon_your_company" > ...
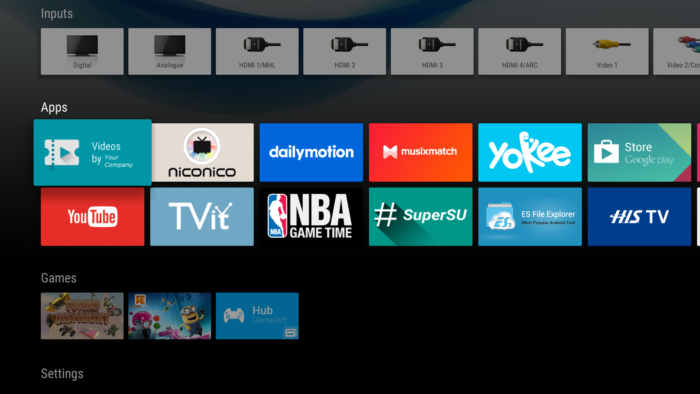
To show application icon in Leanback launcher
アプリのアイコンを表示するには、アプリタグ内に以下を記載。
<application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:theme="@style/Theme.Leanback" > ...
アプリアイコンはActivityのランチャーアイコンとは異なるので注意。アプリアイコンはAndroid TVの設定→アプリ画面で確認できる。
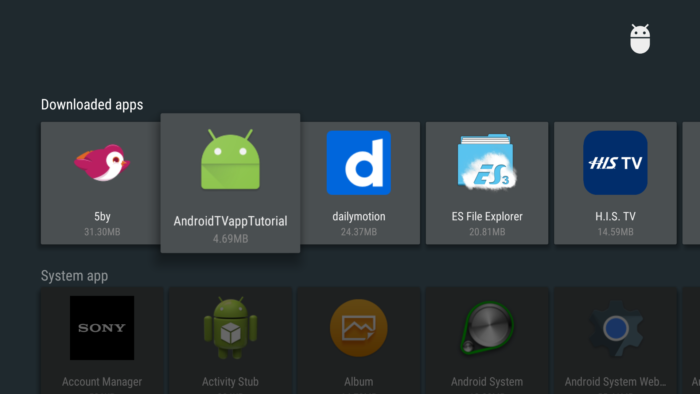
Android TV向けのAndroidManifestの設定に関しては Android developers pageも参照。
次章BrowseFragmentの構成 – Android TVアプリ開発入門2 では BrowseFragment
, HeadersFragment
, RowsFragment
をより深く説明する。Adapter と Presenter を用いて実際にBrowseFragment内にアイテムの表示をしていく。